컨트롤러
BController.java
package com.all.test01.controller;
import javax.servlet.http.HttpServletRequest;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import com.all.test01.command.BCommand;
import com.all.test01.command.BContentCommand;
import com.all.test01.command.BDeleteCommand;
import com.all.test01.command.BListCommand;
import com.all.test01.command.BModifyCommand;
import com.all.test01.command.BReplyCommand;
import com.all.test01.command.BReplyViewCommand;
import com.all.test01.command.BWriteCommand;
@Controller
public class BController {
BCommand command = null;
@RequestMapping("/list")
public String list(Model model) {
System.out.println("list()");
command = new BListCommand();
command.execute(model);
return "list";
}
@RequestMapping("/write_view")
public String write_view(Model model) {
return "write_view";
}
@RequestMapping("/write")
public String write(HttpServletRequest request,Model model) {
model.addAttribute("request",request);
command = new BWriteCommand();
command.execute(model);
return "redirect:/list";
}
@RequestMapping("/content_view")
public String content_view(HttpServletRequest request,Model model) {
model.addAttribute("request",request);
command = new BContentCommand();
command.execute(model);
return "content_view";
}
@RequestMapping("/modify")
public String modify(HttpServletRequest request,Model model) {
model.addAttribute("request",request);
command = new BModifyCommand();
command.execute(model);
return "redirect:/list";
}
@RequestMapping("/delete")
public String delete(HttpServletRequest request,Model model) {
model.addAttribute("request",request);
command = new BDeleteCommand();
command.execute(model);
return "redirect:/list";
}
@RequestMapping("/reply_view")
public String reply_view(HttpServletRequest request,Model model) {
model.addAttribute("request",request);
command = new BReplyViewCommand();
command.execute(model);
return "reply_view";
}
@RequestMapping("/reply")
public String reply(HttpServletRequest request,Model model) {
model.addAttribute("request",request);
command = new BReplyCommand();
command.execute(model);
return "redirect:/list";
}
}
DTO
BDto.java
package com.all.test01.dto;
import java.sql.Timestamp;
public class BDto {
private int bId;
private String bName;
private String bTitle;
private String bContent;
private Timestamp bDate;
private int bHit;
private int bGroup;
private int bStep;
private int bIndent;
public BDto() {
}
public BDto(int bId, String bName, String bTitle, String bContent, java.sql.Timestamp bDate, int bHit, int bGroup, int bStep,
int bIndent) {
this.bId = bId;
this.bName = bName;
this.bTitle = bTitle;
this.bContent = bContent;
this.bDate = bDate;
this.bHit = bHit;
this.bGroup = bGroup;
this.bStep = bStep;
this.bIndent = bIndent;
}
public int getbId() {
return bId;
}
public void setbId(int bId) {
this.bId = bId;
}
public String getbName() {
return bName;
}
public void setbName(String bName) {
this.bName = bName;
}
public String getbTitle() {
return bTitle;
}
public void setbTitle(String bTitle) {
this.bTitle = bTitle;
}
public String getbContent() {
return bContent;
}
public void setbContent(String bContent) {
this.bContent = bContent;
}
public Timestamp getbDate() {
return bDate;
}
public void setbDate(Timestamp bDate) {
this.bDate = bDate;
}
public int getbHit() {
return bHit;
}
public void setbHit(int bHit) {
this.bHit = bHit;
}
public int getbGroup() {
return bGroup;
}
public void setbGroup(int bGroup) {
this.bGroup = bGroup;
}
public int getbStep() {
return bStep;
}
public void setbStep(int bStep) {
this.bStep = bStep;
}
public int getbIndent() {
return bIndent;
}
public void setbIndent(int bIndent) {
this.bIndent = bIndent;
}
}
DAO
BDao.java
package com.all.test01.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Timestamp;
import java.util.ArrayList;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.sql.DataSource;
import com.all.test01.dto.BDto;
public class BDao {
DataSource ds;
// Connection conn;
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
public BDao() {
try {
Context init = new InitialContext();
ds = (DataSource) init.lookup("java:comp/env/jdbc/OracleDB");
connection = ds.getConnection();
System.out.println("DB 연결 성공!");
} catch (Exception ex) {
System.out.println("DB 연결실패 : " + ex);
return;
}
}
public ArrayList<BDto> list() {
System.out.println("아니요");
ArrayList<BDto> dtos = new ArrayList<BDto>();
try {
connection = ds.getConnection();
String query = "select bId, bName, bTitle, bContent, bDate, bHit, bGroup, bStep, bIndent from mvc_board order by bGroup desc, bStep asc";
preparedStatement = connection.prepareStatement(query);
resultSet = preparedStatement.executeQuery();
System.out.println("여기");
while (resultSet.next()) {
System.out.println("여기 탄다");
int bId = resultSet.getInt("bId");
String bName = resultSet.getString("bName");
String bTitle = resultSet.getString("bTitle");
String bContent = resultSet.getString("bContent");
Timestamp bDate = resultSet.getTimestamp("bDate");
int bHit = resultSet.getInt("bHit");
int bGroup = resultSet.getInt("bGroup");
int bStep = resultSet.getInt("bStep");
int bIndent = resultSet.getInt("bIndent");
BDto dto = new BDto(bId, bName, bTitle, bContent, bDate, bHit, bGroup, bStep, bIndent);
dtos.add(dto);
}
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
} finally {
try {
if (resultSet != null)
resultSet.close();
if (preparedStatement != null)
preparedStatement.close();
if (connection != null)
connection.close();
} catch (Exception e2) {
// TODO: handle exception
e2.printStackTrace();
}
}
return dtos;
}
public void write(String bName, String bTitle, String bContent) {
// TODO Auto-generated method stub
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = ds.getConnection();
String query = "insert into mvc_board (bId, bName, bTitle, bContent, bHit, bGroup, bStep, bIndent) values (mvc_board_seq.nextval, ?, ?, ?, 0, mvc_board_seq.currval, 0, 0 )";
preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, bName);
preparedStatement.setString(2, bTitle);
preparedStatement.setString(3, bContent);
int rn = preparedStatement.executeUpdate();
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
} finally {
try {
if (preparedStatement != null)
preparedStatement.close();
if (connection != null)
connection.close();
} catch (Exception e2) {
// TODO: handle exception
e2.printStackTrace();
}
}
}
public BDto detail(int bId) {
BDto board = null;
try {
connection = ds.getConnection();
String query = "select * from mvc_board where bId = ?";
preparedStatement = connection.prepareStatement(query);
preparedStatement.setInt(1, bId);
resultSet = preparedStatement.executeQuery();
if (resultSet.next()) {
int bId2 = resultSet.getInt("bId");
String bName = resultSet.getString("bName");
String bTitle = resultSet.getString("bTitle");
String bContent = resultSet.getString("bContent");
Timestamp bDate = resultSet.getTimestamp("bDate");
int bHit = resultSet.getInt("bHit");
int bGroup = resultSet.getInt("bGroup");
int bStep = resultSet.getInt("bStep");
int bIndent = resultSet.getInt("bIndent");
board = new BDto(bId, bName, bTitle, bContent, bDate, bHit, bGroup, bStep, bIndent);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
if (preparedStatement != null)
preparedStatement.close();
if (connection != null)
connection.close();
} catch (Exception e2) {
// TODO: handle exception
e2.printStackTrace();
}
}
return board;
}
public void modify(BDto dto, String bName, String bTitle, String bContent) {
try {
connection = ds.getConnection();
String query = "update mvc_board set bName = ?, bTitle = ?, bContent = ? where bId = ?";
preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, bName);
preparedStatement.setString(2, bTitle);
preparedStatement.setString(3, bContent);
preparedStatement.setInt(4, dto.getbId());
int rn = preparedStatement.executeUpdate();
System.out.println("수정완료");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
if (preparedStatement != null)
preparedStatement.close();
if (connection != null)
connection.close();
} catch (Exception e2) {
// TODO: handle exception
e2.printStackTrace();
}
}
}
public boolean isBoardWriter(int bId, String bName) {
try {
connection = ds.getConnection();
String query = "select * from mvc_board where bId = ?";
preparedStatement = connection.prepareStatement(query);
preparedStatement.setInt(1, bId);
resultSet = preparedStatement.executeQuery();
if (bName.equals(resultSet.getString("bName"))) {
return true;
}
} catch (SQLException e) {
System.out.println("작성자 에러 : " + e);
} finally {
try {
if (preparedStatement != null)
preparedStatement.close();
if (connection != null)
connection.close();
} catch (Exception e2) {
// TODO: handle exception
e2.printStackTrace();
}
}
return false;
}
public void delete(int bId) {
try {
connection = ds.getConnection();
String query = "delete from mvc_board where bId = ?";
preparedStatement = connection.prepareStatement(query);
preparedStatement.setInt(1, bId);
int rn = preparedStatement.executeUpdate();
System.out.println("삭제완료");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
if (preparedStatement != null)
preparedStatement.close();
if (connection != null)
connection.close();
} catch (Exception e2) {
// TODO: handle exception
e2.printStackTrace();
}
}
}
public void reply_write(int bId, String bName, String bTitle, String bContent) {
// TODO Auto-generated method stub
// Connection connection = null;
// PreparedStatement preparedStatement = null;
try {
connection = ds.getConnection();
String query = "insert into mvc_board (bId, bName, bTitle, bContent, bHit, bGroup, bStep, bIndent) values (mvc_board_seq.nextval, ?, ?, ?, 0, mvc_board_seq.currval, 0, 0 )";
preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, bName);
preparedStatement.setString(2, bTitle);
preparedStatement.setString(3, bContent);
int rn = preparedStatement.executeUpdate();
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
} finally {
try {
if (preparedStatement != null)
preparedStatement.close();
if (connection != null)
connection.close();
} catch (Exception e2) {
// TODO: handle exception
e2.printStackTrace();
}
}
}
public void hitCount(int bId) {
try {
connection = ds.getConnection();
String query = "update mvc_board set bHit = bHit+1 where bId = ?";
preparedStatement = connection.prepareStatement(query);
preparedStatement.setInt(1, bId);
//preparedStatement.setInt(2, bId);
int rn = preparedStatement.executeUpdate();
System.out.println("조회수증가");
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
if (preparedStatement != null)
preparedStatement.close();
if (connection != null)
connection.close();
} catch (Exception e2) {
// TODO: handle exception
e2.printStackTrace();
}
}
}
public void reply(String bId, String bName, String bTitle, String bContent, String bGroup, String bStep, String bIndent) {
// TODO Auto-generated method stub
// Connection connection = null;
// PreparedStatement preparedStatement = null;
try {
connection = ds.getConnection();
String query = "insert into mvc_board (bId, bName, bTitle, bContent, bGroup, bStep, bIndent) values (mvc_board_seq.nextval, ?, ?, ?, ?, ?, ?)";
preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, bName);
preparedStatement.setString(2, bTitle);
preparedStatement.setString(3, bContent);
preparedStatement.setInt(4, Integer.parseInt(bGroup));
preparedStatement.setInt(5, Integer.parseInt(bStep) + 1);
preparedStatement.setInt(6, Integer.parseInt(bIndent) + 1);
int rn = preparedStatement.executeUpdate();
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
} finally {
try {
if(preparedStatement != null) preparedStatement.close();
if(connection != null) connection.close();
} catch (Exception e2) {
// TODO: handle exception
e2.printStackTrace();
}
}
}
}
command
BCommand(인터페이스)
package com.all.test01.command;
import org.springframework.ui.Model;
public interface BCommand {
void execute(Model model);
}
BContentCommand.java
package com.all.test01.command;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.ui.Model;
import com.all.test01.dao.BDao;
import com.all.test01.dto.BDto;
public class BContentCommand implements BCommand {
@Override
public void execute(Model model) {
Map<String, Object> map = model.asMap();
HttpServletRequest request = (HttpServletRequest) map.get("request");
int bId =Integer.parseInt(request.getParameter("bId"));
BDao dao = new BDao();
BDto dto = new BDto();
dao.hitCount(bId);
dto = dao.detail(bId);
model.addAttribute("content_view",dto);
}
}
BDeleteCommand.java
package com.all.test01.command;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.ui.Model;
import com.all.test01.dao.BDao;
public class BDeleteCommand implements BCommand {
@Override
public void execute(Model model) {
Map<String, Object> map = model.asMap();
HttpServletRequest request = (HttpServletRequest) map.get("request");
int bId = Integer.parseInt(request.getParameter("bId"));
BDao dao = new BDao();
dao.delete(bId);
}
}
BListCommand.java
package com.all.test01.command;
import java.util.ArrayList;
import org.springframework.ui.Model;
import com.all.test01.dao.BDao;
import com.all.test01.dto.BDto;
public class BListCommand implements BCommand {
@Override
public void execute(Model model) {
BDao dao = new BDao();
ArrayList<BDto> dtos = dao.list();
model.addAttribute("list",dtos);
}
}
BModifyCommand.java
package com.all.test01.command;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.ui.Model;
import com.all.test01.dao.BDao;
import com.all.test01.dto.BDto;
public class BModifyCommand implements BCommand {
@Override
public void execute(Model model) {
Map<String, Object> map = model.asMap();
HttpServletRequest request = (HttpServletRequest) map.get("request");
BDao dao = new BDao();
BDto dto = new BDto();
int bId = Integer.parseInt(request.getParameter("bId"));
String bName = request.getParameter("bName");
String bTitle = request.getParameter("bTitle");
String bContent = request.getParameter("bContent");
/*
boolean usercheck = dao.isBoardWriter(bId,bName);
if(usercheck == false) {
System.out.println("수정할 권한이 없습니다!");
} else {
dto = dao.detail(bId);
dao.modify(dto,bName, bTitle, bContent);
}
*/
dto = dao.detail(bId);
dao.modify(dto,bName, bTitle, bContent);
}
}
BReplyCommand.java
package com.all.test01.command;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.ui.Model;
import com.all.test01.dao.BDao;
public class BReplyCommand implements BCommand {
@Override
public void execute(Model model) {
Map<String, Object> map = model.asMap();
HttpServletRequest request = (HttpServletRequest) map.get("request");
String bId = request.getParameter("bId");
String bName = request.getParameter("bName");
String bTitle = request.getParameter("bTitle");
String bContent = request.getParameter("bContent");
String bGroup = request.getParameter("bGroup");
String bStep = request.getParameter("bStep");
String bIndent = request.getParameter("bIndent");
BDao dao = new BDao();
dao.reply(bId, bName, bTitle, bContent, bGroup, bStep, bIndent);
}
}
BReplyViewCommand.java
package com.all.test01.command;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.ui.Model;
import com.all.test01.dao.BDao;
import com.all.test01.dto.BDto;
public class BReplyViewCommand implements BCommand {
@Override
public void execute(Model model) {
Map<String, Object> map = model.asMap();
HttpServletRequest request = (HttpServletRequest) map.get("request");
int bId =Integer.parseInt(request.getParameter("bId"));
BDao dao = new BDao();
BDto dto = new BDto();
dto = dao.detail(bId);
model.addAttribute("reply_view",dto);
}
}
BWriteCommand.java
package com.all.test01.command;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.springframework.ui.Model;
import com.all.test01.dao.BDao;
public class BWriteCommand implements BCommand {
@Override
public void execute(Model model) {
// TODO Auto-generated method stub
Map<String, Object> map = model.asMap();
HttpServletRequest request = (HttpServletRequest) map.get("request");
String bName = request.getParameter("bName");
String bTitle = request.getParameter("bTitle");
String bContent = request.getParameter("bContent");
BDao dao = new BDao();
dao.write(bName, bTitle, bContent);
}
}
결과
리스트 페이지
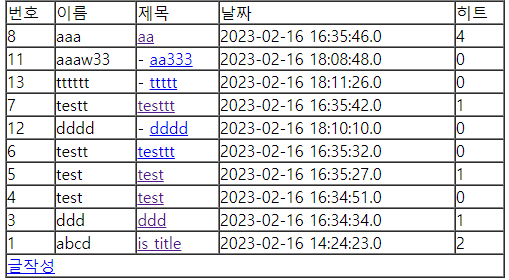
상세페이지
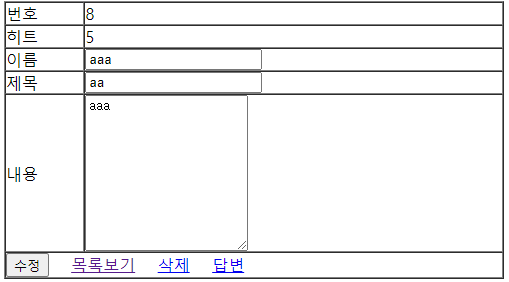
답변페이지
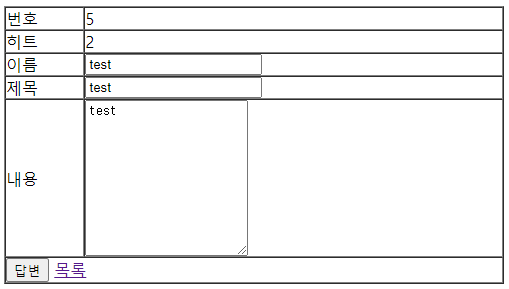
'Spring' 카테고리의 다른 글
[스프링] 프로젝트 생성 기본경로(url) 변경 (0) | 2023.03.02 |
---|---|
[스프링] 스프링 JdbcTemplate 사용하기 (0) | 2023.02.17 |
[스프링] Validator를 이용한 서버 유효성 검사 (0) | 2023.02.16 |
[스프링 에러] An internal error occurred during: "Requesting Java AST from selection".com.ibm.icu.text.UTF16.isSurrogate(C)Z (1) | 2023.02.13 |
[스프링 에러] @PostConstruct @PreDestroy 에러 빨간 밑줄 (0) | 2023.02.13 |